Responsive HTML Email Template From Scratch Tutorial
Responsive HTML email template design and development can seem challenging when your first getting started on your email development journey, but with just a handful of key elements to HTML email you can start designing your own templates within hours. In this tutorial I’ll show you all of the key elements to HTML email development by assembling the knowledge-base here at Responsive HTML Email.
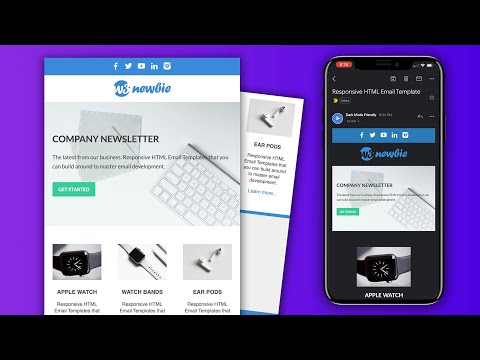
HTML Email Doctype
Let’s first start with our email doctype for our template since this is the very first part of the HTML document we’ll be building inside of for our email design. If you need more guidance on creating an HTML document and getting started with a text editor, please see the resources page where there is an introduction to the Visual Studio Code Text Editor. In HTML email, the simple answer to “what is the best email doctype?” is whichever one works in the most email clients (such as Outlook).
Some may argue that the HTML5 doctype is OK to use for email, but the best testing service for email designs (Litmus & Email On Acid) still recommend XHTML Email Doctype as it allows for proper rending on all email clients. Here is the complete document type HTML with the xml namespace link included for the xlmns attribute in addition to language english:
<!DOCTYPE htmlPUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" lang="en">
<head>
...
</head>
<body>
...
</body>
</html>
HTML Email Head Metadata
Now that we have our doctype sorted out, let’s direct our focus to the header of the HTML document. The header includes meta tags, which are similar to those used in the latest web development practices, to tell the browser or email client how we want our content to display.
First we’ll declare the character set of the document which is “UTF 8” or the standard character set for numbers and letters on the internet (and in email).
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
Now we’ll add a compatibility meta tag for the rendering of Internet Explorer and Microsoft Edge web browsers:
<meta http-equiv="X-UA-Compatible" content="IE=edge">
Next we’ll control the layout on mobile browsers and to make it so the scale is set to the zoom of 1 or 100% when the email template is seen on mobile:
<meta name="viewport" content="width=device-width, initial-scale=1.0">
The last set of meta tags included in the head of the document is for dark mode which lets dark mode compatible email clients and web browsers know that it can choose from the light or dark version of the template based on the user’s preference. Learn more about Dark Mode in HTML Email here.
<meta name="color-scheme" content="light dark">
<meta name="supported-color-schemes" content="light dark">
If you plan to have your HTML Email Template display on the web, it’s a good idea to include a title tag for the user to see and for the page to be bookmarked in a users browser.
<title>HTML Email Template Project</title>
CSS in HTML Email
Some of the older clients still require inline styling with CSS in HTML email such as Microsoft Outlook in addition to the use of conditional statements for full compatibility, but with modern HTML email clients (Gmail, Apple Mail) we can take care of some of the styling in an internal style sheet.
Internal CSS Styling is included in the <head> section of the document using the <style> tag along with media queries for responsive-specific CSS and Dark Mode.
<style type="text/css">
...
</style>
For this design, we’ll start with adding the internal CSS style above in the head section after the meta tags and title.
HTML Email Media Queries
Media queries allow us to apply specific CSS styling to our HTML depending on the width we set for the media query. This will work in modern email clients but not in Outlook where we’ll need our inline CSS.
Our email design will have two different breakpoints, at 600px and 400px, which will use the “max-width” property. Using maximum-width in our media query means the HTML email css styling added inside of it will be applied under these set widths from the full width *or* up-to the set width starting from 0px. So, under 600px the first styles will be applied, then under 400px. Here are the media queries that will be added inside of the <style> tag that already sits in the <head> section:
@media only screen and (max-width: 600px) {...}
@media only screen and (max-width: 400px) {...}
We’ll reference these later in the tutorial as we add our styling.
HTML Email Body
The HTML email body or <body> tag in our HTML email document is the start of setting up our document’s appearance in email clients and web browsers. We’ll need to strip some inherent styling from the document in order to use up the full client or browser window as our canvas. We don’t need to do anything with the <body> tag itself which is already part of the doc but we’ll add the following style in the internal style sheet:
body {
Margin: 0;
padding: 0;
background-color: #f6f9fc;
}
The margin and padding being set to zero does away with any spacing to the edges of email clients and browsers the template design may have otherwise. For best practice, capitalize the “M” in Margin for proper rendering of Windows email clients such as Outlook. You’ll also notice we’ve added a background color here, this is for the body outside of the template which we’ll reinforce later in the tutorial.Following suit with the potential <body> spacing issues, we’ll do the same in removing padding and border where it applies for all of our table, td (table data), and image HTML tags as follows:
table {
border-space: 0;
}
td {
padding: 0;
}
img {
border: 0;
}
This includes all of our “reset” styling for the template and we’re ready to get started inside of the HTML body.
HTML Email Width & Background Color
Let’s get started with centering our HTML email template. This is going to set up our table’s width and solidify it inside of email clients while still allowing for responsiveness. We’ll start this section with a “wrapper”. In website design and development a wrapper is usually the term for setting a max width but in our email template it will be used similar to the typical <body> styling. The reason for this is because we need to reinforce the background color and width in case email clients don’t respect the body styling. We’ll do this with “width 100%” and “background-color: #f6f9fc;” by adding it to the <center class=”wrapper”> element. Yes, “center” is a very old HTML tag for those of us familiar with the progression of the mark-up language but it still applies in HTML email.
Then, in addition to the width and background color, we’ll need to set up the table inside of the element with ”table-layout: fixed;” to make sure the table can’t span any wider than the wrapper. Also, I’ll be adding a little bit of padding to the bottom of the design because it looks cool:
<center class=”wrapper”>
.wrapper{
width:100%;
background-color:#f6f9fc;
table-layout:fixed;
padding-bottom:40px;
}
Lastly, for the width and centering to set up our table, it’s best to reinforce the maximum width of the main table we’ll be adding and the background color of the template by using a simple <div> element (the only div in the design). I’ll name is “outer” as a reminder that is just outside of the template table as a parent element:
<div class=”outer”>
.outer {
max-width: 600px;
background-color: #ffffff;
}
HTML Email Preheader Text
HTML email preheader text is the preview text of the email message that is seen underneath or next to the title of the email subject when it’s received in the inbox. Email service providers such as MailChimp allow you to add preheader, or preview text, within their email builder features, but in HTML email we use the raw code to add it! This will show our email preview text in all email clients, including preview text for Outlook.
For best practice, keep the length of the preheader/preview text between 40 characters and 130 characters with the sweet spot around 85-100 characters. This makes sure that the message is short enough to be seen on small devices such as cell phones, and takes up the allotted space on full-width desktop clients.
Now for the HTML and CSS. The purpose of our code here for an email preview is to make sure it isn’t seen in our design, so just beneath and inside of the <div class=”wrapper”> and <div class=”outer”> tags which set the background colors and width of our template to set up our table.
<div style=”font-size: 0px; line-height: 1px; mso-line-height-rule: exactly; display: none; max-width: 0px; max-height:0px; opacity: 0; overflow:hidden; mso-hide: all;”>
<!– Add 85-100 Characters of Preheader Text Here –>
</div>
Centering the HTML Email Template Table
To center the HTML email we’ll need to add our first and main table inside of our “wrapper” center tag and “outer” div tag elements mentioned above when setting the width. To do this we’ll use a classic <table> tag which is still needed for proper email client rendering (no we can’t use divs) and we’ll give our table a class called “main” in addition to reinforcing the centering of the template with the align=”center” attribute as follows:
<table class=”main” align=”center”>
Now that we have the “center” value added to the align attribute, we can add some more centering! This was email clients have no choice but to center our template. Additionally, we’ll give this element a width of 100% so our template takes up the full width on devices under 600px, add a maximum width of 600px to make sure that the design doesn’t span any wider, and set the border spacing to “0” so no unwanted space appears around the edges of the table:
.main {
Margin: 0 auto;
width: 100%;
max-width: 600px;
border-spacing: 0;
font-family: sans-serif;
color: #4a4a4a;
}
Feel free to change the font styling and the color of the template fonts to your own styling for the main table.
Social Media Icon Email Template Header
Now it’s time to start adding content inside of our main email template table in form of an email template header that will contain our social media icons. To start this section off, we’ll need to add a new table row. Each main section of the template will always start with the same <tr> table row tag followed by a table data <td> tag that may differ depending on the section.
<tr>
<td>
...
</td>
</tr>
For the email template header, we’ll need to be able to insert some padding around the social media icon images that email clients will apply so in addition to the first set of table row & table data tags, we’ll need to add a table with a new set of these tags inside of it. The inner <td> tag is where we can add the padding to the header, instead of the first one above which some email clients would ignore. This also gives us the opportunity to add a background color to the header and center our images as text since they’ll be inside of links:
<table width="100%">
<tr>
<td style="padding: 10px; background-color: #388CDA; text-align: center;">
...
</td>
</tr>
</table>
Now we can add the social media icon images inside of the table data tag that has the styling set:
<a href=”#”><img src=”img/facebook.png” width=”30″ alt=””></a>
<a href=”#”><img src=”img/twitter.png” width=”30″ alt=””></a>
<a href=”#”><img src=”img/youtube.png” width=”30″ alt=””></a>
<a href=”#”><img src=”img/linkedin.png” width=”30″ alt=””></a>
<a href=”#”><img src=”img/instagram.png” width=”30″ alt=””></a>

HTML Email Template Logo Section
The logo section of the template requires the same structure of HTML tags leading up to the logo image since it will also require some padding around the image:
<tr>
<td>
<table width="100%">
<tr>
<td style="padding: 10px; text-align: center;">
<a href=”#”><img src=”img/logo.png” width=”180″ alt=””></a>
</td>
</tr>
</table>
</td>
</tr>

HTML Email Banner Image
The HTML email banner image requires less coding than the previous two sections. The reason for this is because we don’t need any spacing or styling around the banner image as will take up the full width of the template. We need to add a set width to the banned image with the “width” attribute and a value of 600, for 600px, to have it contained inside of the template’s full width. To make the image contained in the template at screen width’s less than 600px, we’ll add a max-width of 100% as follows:
<tr>
<td>
<a href=”https://w3newbie.com”><img src=”img/1200×700.png” width=”600″ alt=”Banner” style=”max-width: 100%;”></a>
</td>
</tr>
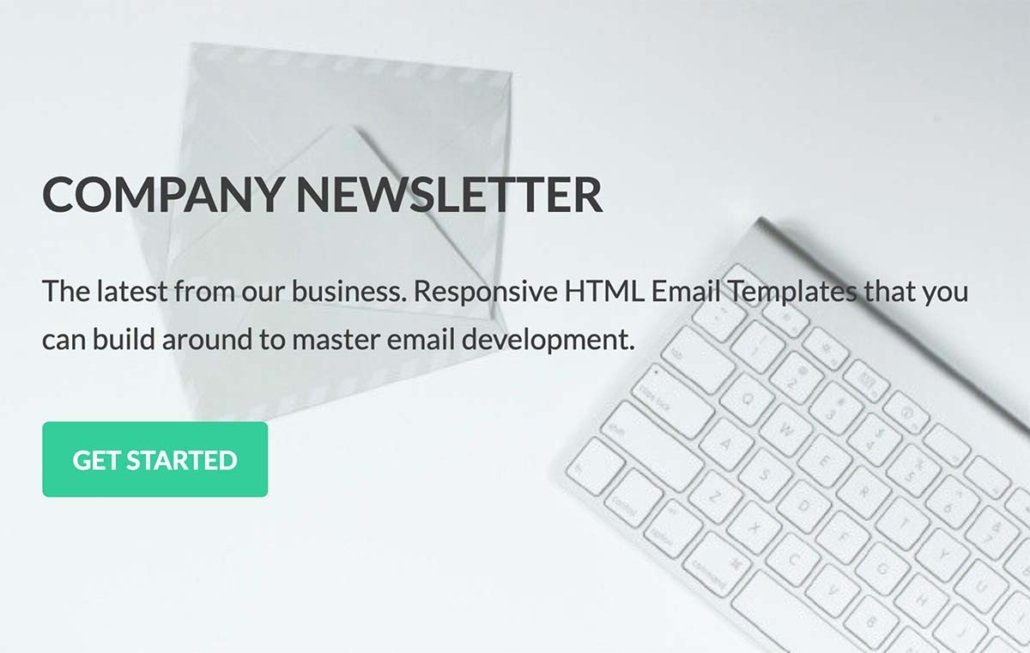
Responsive HTML Email Tables: 3 Column Layout
The meat and potatoes of our design comes in the form of a 3 column html email template layout. This is when some folks may think they are beginning to get lost if they haven’t felt this way already about tables. There are only a few different elements and lines of CSS that make up our responsive table columns though.
We’ll start our three column html email layout off similar to the previous sections of the template where we added styling to images (the social icons and logo). Only this time, instead of adding styling to the second <td> table data tag, we’re going to give the element a class called “three-columns” to let us know this will be containing our individual columns:
<tr>
<td>
<table width="100%">
<tr>
<td class="three-columns">
The “three-columns” table data tag will span the full width of it’s parent table. Now we can start to style this section using the class. Since all of our content will be centered, we can use the ‘text-align: center;’ style declaration to position all of our content in the center. Then we’ll add some spacing to the top and bottom of the section to make it so the columns we’ll add will be separated from the template banner image and footer section. Lastly, we’ll need to apply ‘font-size: 0;’ because the inherit font size that is applied to <td> tags will later make it so our 200px columns can’t align next to each other without this applied. Here is our CSS:
.three-columns {
text-align: center;
padding-top: 40px;
padding-bottom: 30px;
font-size: 0;
}
Now let’s begin to add our first of three email columns. Since our “three-columns” element is a table data tag, we’ll start a new <table> inside of it which we’ll name “column” as it represents one of three columns. It’s also important that we create a <td> tag inside of the table that we can apply spacing to in the form of padding so our column content isn’t bunched up to the edge of the allotted 200px width. To do this, we’ll include a new table row and table data tag which is name “padding” as it’s class:
<table class="column">
<tr>
<td class="padding">
Here come’s the responsive HTML email multi-column magic. We’re ready for the column’s CSS styling. First we’ll add a width of 100% to make sure the table column will display at the full width of the parent element. Then we’ll set the max-width to 200px so all three of our columns can display inside of the 600px layout. To get the columns displaying inline we’ll use the style declaration ‘display: inline-block;’ and to make the columns stack on top of one another for responsiveness we’ll used the ‘vertical-align: top;’ styling. For padding, we’ll apply 15px of space all around to the inside of our columns. Here is the CSS:
.three-columns .column {
width: 100%;
max-width: 200px;
display: inline-block;
vertical-align: top;
}
.padding {
padding: 15px;
}
We’re ready to add our column content now. The content can include anything you want to add to your template as long as you have it set inside of a new table and set of table row <tr> & table data <td> tags as follows:
<table class="content">
<tr>
<td>
There is only one problem with this section now, we won’t be able to see any of the text we add because of the ‘font-size: 0;’ style declaration that is making it so our 200px width tables can all be inline. To correct this, apply the following font-size styling to the ‘content’ class with an optional line-height adjustment for more spacing around the text:
.content {
font-size: 15px;
line-height: 20px;
}
This template design has an image on top of the text and link for the content inside of the column. Here is the full HTML starting from the “content” class as mentioned above:
<table class="content">
<tr>
<td>
<a href="#"><img src="img/380x280.jpg" alt="" width="150" style="max-width: 150px;" class="third-img"></a>
</td>
</tr>
<tr>
<td style="padding: 10px;">
<p style="font-weight: bold; font-size: 17px;">APPLE WATCH</p>
<p>Responsive HTML Email Templates that you can build around to master email development.</p>
<a href="#">Learn more..</a>
</td>
</tr>
</table>
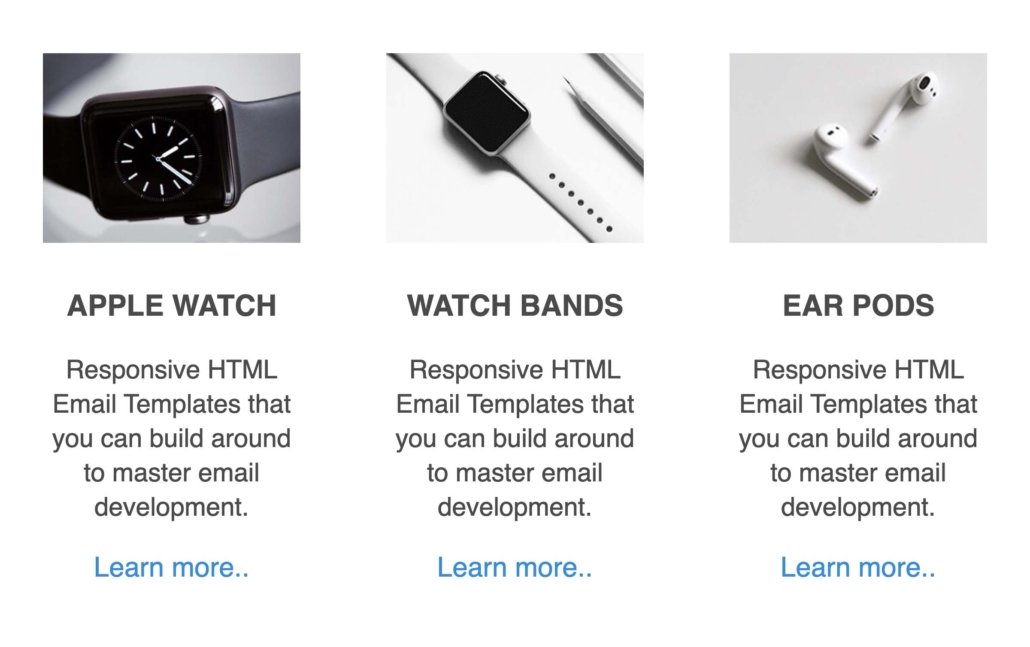
You might notice that a class has been added for the image called “third-img”. This class will be referenced in the CSS media query for screen widths underneath 400px effecting both the first and second of the three columns. So, repeat this first column for the second column HTML. For the third column, we’ll use a different class name. This time it will be “third-img-last” and this will be referenced in the 600px width media query. What is the purpose of these classes? When the screen width drops underneath 600px two of our columns will be inline and the third will sit underneath the first two which will be centered. So we’ll reference the “third-img-last” class to change with 150px width of the image to 200px and remove the padding on the left & right which was added using the “padding” class. Here is the media query:
@media screen and (max-width: 600px) {
img.third-img-last {
width: 200px!important;
max-width: 200px!important;
}
.padding {
padding-left: 0!important;
padding-right: 0!important;
}
}
The first two images will have the same width of 200px applied on screens narrower than 400px using the “third-img” class:
@media screen and (max-width: 400px) {
img.third-img {
width: 200px!important;
max-width: 200px!important;
}
}
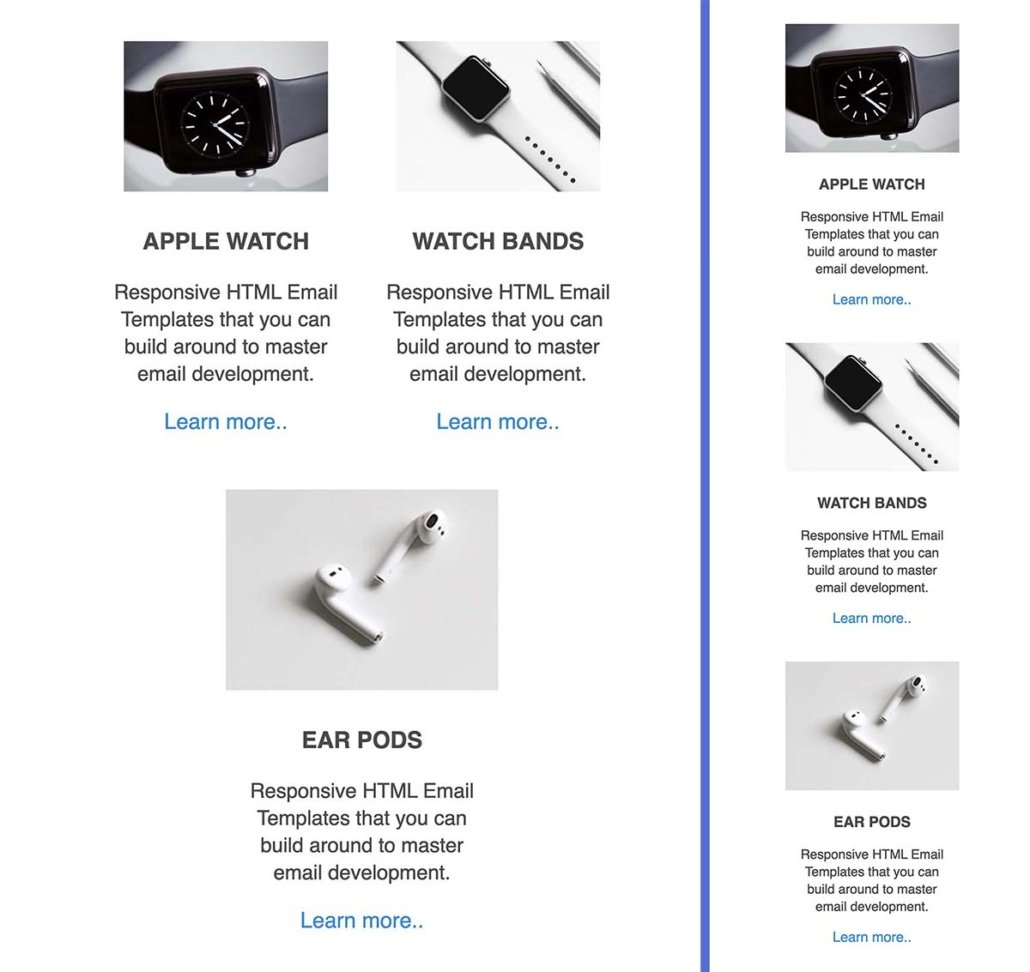
HTML Email Footer Section
The rest of the template design is a breeze. Before and after the email template footer, there will be a simple border spacer matching the header background color of the template, then inside of the footer a light gray background color will be applied and images, text, and links will be added inside of it. Here is the full remaining HTML for the footer section of the template:
<tr>
<td style="background-color: #efefef;">
<table width="100%">
<tr>
<td style="padding: 20px; text-align: center; padding-bottom:10px;">
<a href="#"><img src="img/w3newbie.png" width="160" alt=""></a>
<p style="font-size: 16px; Margin-top: 18px; Margin-bottom: 10px;">w3newbie HTML Email</p>
<p style="font-size: 16px; Margin-top: 18px; Margin-bottom: 10px;">123 Street Road, City, State 55555</p>
<p><a href="mailto:email@email.com">email@email.com</a></p>
<p><a href="tel:18008888888">1-800-888-8888</a></p>
<p style="Margin-top: 38px; Margin-bottom: 30px;"><a href="#">SUBSCRIBE!</a></p>
</td>
</tr>
</table>
</td>
</tr>
<tr>
<td style="background-color: #378BDA; border-radius: 0 0 15px 15px;" height="20"></td>
</tr>
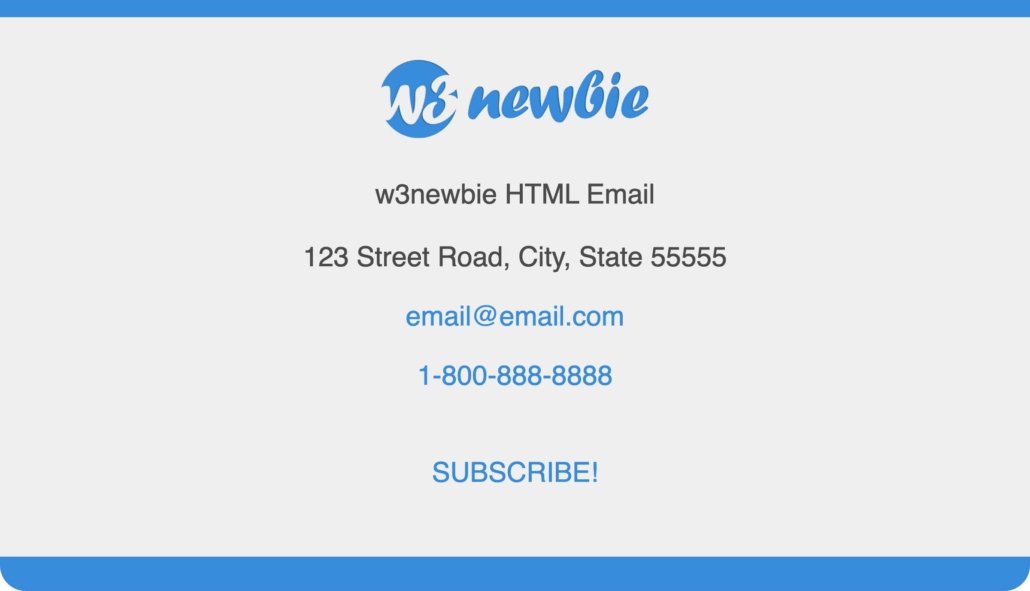
That concludes our full Responsive HTML Email Template Tutorial! Be sure to subscribe and check out the HTML Email Mastery Course, thanks for reading!